Terraform is an open-source Infrastructure as Code (IaC) tool developed by HashiCorp that enables users to define, provision, and manage cloud infrastructure through human-readable configuration files. It has become an essential tool for DevOps teams across the globe. In this blog post, we will dive into what Terraform is, when to use it, its capabilities, the language it uses with code examples, the Terraform Registry, modules, and other advanced capabilities.
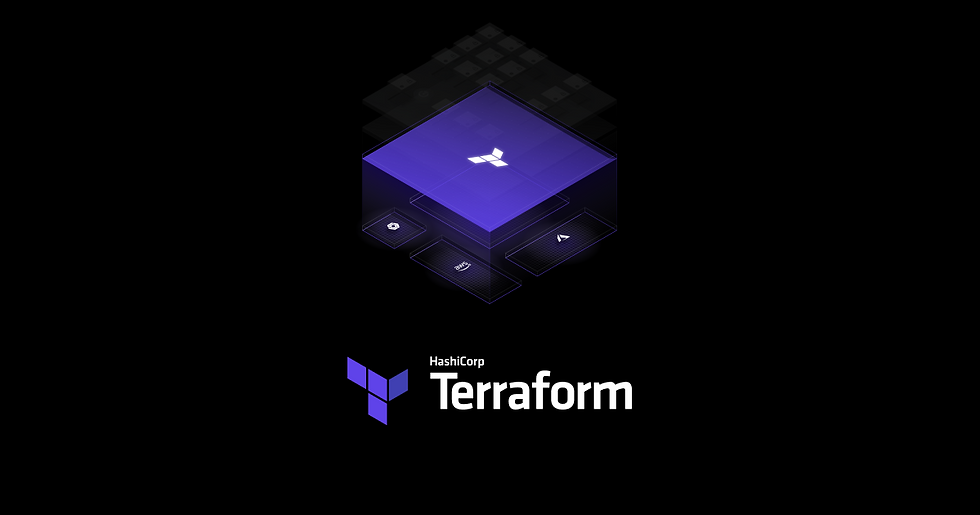
What is Terraform?
Terraform is an IaC tool that allows you to describe and manage your infrastructure using a declarative language called HashiCorp Configuration Language (HCL). It enables you to manage resources in a cloud-agnostic manner, supporting various cloud providers such as AWS, Azure, and Google Cloud Platform. Terraform provides a consistent way to manage cloud resources, ensuring that your infrastructure is always in the desired state.
When to use Terraform?
You should use Terraform when:
You need to manage infrastructure in a consistent, repeatable, and version-controlled way.
You are working with multiple cloud providers and want a unified way to manage your resources.
You want to automate the provisioning and management of your infrastructure.
You need to collaborate with team members on infrastructure management and avoid manual errors.
Capabilities
Terraform boasts several powerful capabilities:
Multi-cloud support: Terraform supports many cloud providers, enabling you to manage a diverse set of resources across different platforms.
Modularity: Terraform allows you to create reusable modules for common infrastructure patterns, improving maintainability and consistency.
Plan and apply: Terraform generates an execution plan that outlines the changes to be made, allowing you to review them before actually applying the changes.
Version control: Terraform configuration files can be versioned and stored in a repository, facilitating collaboration and keeping track of infrastructure changes.
HashiCorp Configuration Language (HCL) and Code Examples
HCL Blocks
In HCL (HashiCorp Configuration Language), there are several types of blocks that serve different purposes within a Terraform configuration. Some of the most common block types are:
1. provider: The provider block is used to configure the backend API connection and credentials for a specific cloud or infrastructure provider (e.g., AWS, GCP, Azure). This block allows Terraform to interact with the provider's API to create, manage, and modify resources.
provider "aws" {
region = "us-west-2"
access_key = "your_access_key"
secret_key = "your_secret_key"
}
2. resource: The resource block is used to define and manage a specific infrastructure object, such as a virtual machine, a network interface, or a database instance. It describes the desired state of the infrastructure object and its required attributes.
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
}
3. data: The data block is used to fetch information about existing resources or configuration data from a provider. This data can then be used to inform the creation or modification of other resources in your configuration.
data "aws_ami" "ubuntu" {
most_recent = true
filter {
name = "name"
values = ["ubuntu/images/hvm-ssd/ubuntu-focal-20.04-amd64-server-*"]
}
owners = ["099720109477"] # Canonical
}
4. variable: The variable block is used to declare input variables that can be referenced throughout the configuration. Input variables make Terraform configurations more flexible and reusable, allowing users to provide different values for different environments or use cases.
variable "region" {
default = "us-west-2"
}
5. output: The output block is used to define values that should be displayed as output after Terraform has applied the configuration. Outputs can be used to provide information about created resources, such as IP addresses, URLs, or resource IDs.
output "instance_public_ip" {
value = aws_instance.example.public_ip
}
6. module: The module block is used to reference and include reusable modules within your configuration. Modules are self-contained packages of Terraform configurations that can be shared across multiple projects or environments.
module "vpc" {
source = "terraform-aws-modules/vpc/aws"
version = "2.77.0"
name = "my-vpc"
cidr = "10.0.0.0/16"
azs = ["us-west-2a", "us-west-2b", "us-west-2c"]
private_subnets = ["10.0.1.0/24", "10.0.2.0/24", "10.0.3.0/24"]
public_subnets = ["10.0.101.0/24", "10.0.102.0/24", "10.0.103.0/24"]
}
7. locals: The locals block is used to define local values or expressions that can be referenced within the configuration. Local values can help simplify complex expressions, reuse common values, or perform computations based on input variables or data sources.
locals {
common_tags = {
Terraform = "true"
Environment = "dev"
}
}
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
tags = merge(local.common_tags, {
Name = "example-instance"
})
}
8. terraform: The terraform block is used to configure settings related to the Terraform runtime itself, such as the required Terraform version, backend configuration for remote state storage, or required providers and their versions.
terraform {
required_version = ">= 0.14"
backend "s3" {
bucket = "my-terraform-state"
key = "terraform.tfstate"
region = "us-west-2"
}
}
These different block types work together to create a complete Terraform configuration, defining the desired state of the infrastructure, the providers to interact with, and the necessary input and output values. By using these blocks, you can create flexible, modular, and reusable configurations to manage your infrastructure efficiently and effectively.
Here are some examples of HCL code:
1. Basic AWS EC2 instance:
provider "aws" {
region = "us-west-2"
}
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
tags = {
Name = "example-instance"
}
}
In this example, we define a provider block for AWS, specifying the region where our resources will be provisioned. The resource block is used to create an EC2 instance with the specified Amazon Machine Image (AMI) ID and instance type. Additionally, we assign a tag to the instance with a key of "Name" and a value of "example-instance".
2. Variable usage
variable "region" {
default = "us-west-2"
}
variable "instance_type" {
default = "t2.micro"
}
provider "aws" {
region = var.region
}
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = var.instance_type
tags = {
Name = "example-instance"
}
}
In this example, we introduce variables to make the configuration more flexible and reusable. We define two variables, region and instance_type, with default values of "us-west-2" and "t2.micro", respectively. These variables are then referenced in the provider and resource blocks using the var.<variable_name> syntax. By doing so, we can easily change the region or instance type without modifying the actual resource or provider configuration.
HCL's syntax and structure make it an ideal language for defining infrastructure resources. It offers a concise and intuitive way to declare the desired state of resources and makes it easy to understand the infrastructure being managed. Overall, HCL's design contributes significantly to the user-friendly nature of Terraform, making it a popular choice for Infrastructure as Code.
Terraform Registry and Modules
The Terraform Registry (https://registry.terraform.io/) is a centralized repository of publicly available Terraform modules and providers. It simplifies the process of discovering and sharing reusable infrastructure components. Modules are self-contained, reusable packages that can be versioned and shared across teams.
An example of using a module from the Terraform Registry:
module "vpc" {
source = "terraform-aws-modules/vpc/aws"
version = "2.77.0"
name = "my-vpc"
cidr = "10.0.0.0/16"
azs = ["us-west-2a", "us-west-2b", "us-west-2c"]
private_subnets = ["10.0.1.0/24", "10.0.2.0/24", "10.0.3.0/24"]
public_subnets = ["10.0.101.0/24", "10.0.102.0/24", "10.0.103.0/24"]
enable_nat_gateway = true
single_nat_gateway = false
enable_vpn_gateway = false
enable_dns_hostnames = true
}
In this example, we are using the "terraform-aws-modules/vpc/aws" module from the Terraform Registry to create a VPC with private and public subnets, NAT gateways, and other configurations. The source attribute specifies the module's location, while the version attribute pins the module to a specific version.
Advanced Terraform Features In addition to the previously discussed capabilities, Terraform offers several advanced features that can further enhance your infrastructure management experience:
Workspaces: Terraform workspaces allow you to maintain separate instances of your infrastructure for different environments (e.g., development, staging, production). This enables you to manage environment-specific configurations without duplicating code.
State management: Terraform keeps track of your infrastructure's state, which is crucial for determining the differences between the desired and actual infrastructure. Advanced state management features, such as remote state storage and state locking, provide additional security and collaboration benefits.
Provisioners: Terraform provisioners enable you to execute scripts on your resources during the provisioning or destruction process. While not recommended for regular use, they can be helpful for specific use cases where other solutions might not be applicable.
Importing existing resources: Terraform can import existing resources into your configuration, allowing you to manage them alongside newly provisioned infrastructure. This helps you integrate Terraform into your existing environments without starting from scratch.
Sentinel Policy as Code: With the Sentinel integration (available in Terraform Enterprise), you can enforce policies across your infrastructure using a policy as code approach. This ensures compliance with organizational and regulatory requirements.
Terraform Cloud and Enterprise: Terraform Cloud and Enterprise offer additional collaboration, governance, and automation features, such as remote state management, private module registry, role-based access control, and more. These offerings are particularly valuable for larger teams and organizations.
Conclusion
In conclusion, Terraform is a powerful Infrastructure as Code tool that simplifies the management of cloud infrastructure across multiple providers. By using its declarative language (HCL), you can create and manage infrastructure in a consistent, repeatable, and version-controlled manner. The Terraform Registry provides a vast collection of modules, allowing you to reuse and share infrastructure components easily. With advanced features like workspaces, state management, provisioners, and the integration of Sentinel Policy as Code, Terraform offers an extensive suite of capabilities to help you manage your infrastructure efficiently and effectively.
Whether you are a DevOps engineer, a cloud architect, or just someone who wants to automate infrastructure provisioning and management, Terraform is a must-have tool in your arsenal. With a strong community and continuous improvements, Terraform continues to evolve and adapt to the ever-changing landscape of cloud infrastructure management.
Comments